My kid likes traffic lights. So I decided to quickly build one. I started off on a breadboard with a bunch of components:
- 3 × LEDs (red, amber, green)
- 3 × 470 Ω Resistors (only had two, so the third are actually 2 1 kΩ in parallel)
- 1 × 10 kΩ Resistor
- 1 × Pushbutton Switch
- 1 × Arduino Nano (actually a clone)
The circuitry is relatively simple. All LEDs’ cathodes point to ground (GND) through a 470 Ω resistor each to limit current and not blow anything. The anodes are connected individually to the digital port D3 through D5.
The button works such that, if open, it connects digital port D2 via a 10 kΩ resistor to the 3.3 V output of the Nano. When it closes, it’s supposed to pull D2 to GND, so it’s directly connected to it. The resistor serves to limit the current and – again – not blow anything.
That’s it. Now, for example, pulling D3 high (i.e. applying 3.3 V) will switch on the red LED. Likewise for D4 on the yellow and D5 on the green LED. Let’s introduce some human readable names instead of D2 through D5:
#include <Arduino.h>
#define BUTTON D2
#define LED_RED D3
#define LED_YELLOW D4
#define LED_GREEN D5
In the setup() function, I set the button pin as an input and the LED pins as outputs:
void setup() {
pinMode(LED_RED, OUTPUT);
pinMode(LED_YELLOW, OUTPUT);
pinMode(LED_GREEN, OUTPUT);
pinMode(BUTTON, INPUT);
}
To control the traffic light logic, I scribbled a Moore machine on a piece of paper, starting with a pedestrian traffic light (only red and green).
The machine starts in the red-light state. It remains there, unless the button is pressed. Then, it instantly switches to green (a pedestrian’s dream) where it remains until the button is pressed again.
This behaviour can be implemented by putting a simple switch case statement in the main loop() and implementing a state variable:
// States
enum states{ ST_R,
ST_G
}
void setState(bool red, bool green) {
digitalWrite(LED_RED, red ? HIGH : LOW);
digitalWrite(LED_GREEN, green ? HIGH : LOW);
}
void setRed() { setState(true, false); }
void setGreen() { setState(false, true); }
void loop() {
switch(machineState) {
case ST_R:
// Serial.write("State: Red");
setRed();
break;
case ST_G:
// Serial.write("State: Green");
setGreen();
break;
}
Why use this enum thingy, you say? You could just use an int and call your states 0, 1, 2, etc., you say? Yes, you could also sign up for the biannual global brainfuck contest.
But, hey, wait, there are no transitions!
Exactly! For that purpose I’ll use an interrupt.
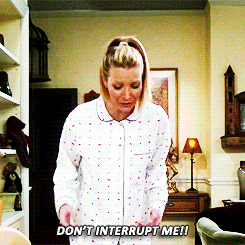
Just add one more function:
void ISR_ButtonToggle() {
Serial.write("BUUUUTTON!\n");
if(machineState == ST_R) {
machineState = ST_G;
} else if (machineState == ST_G) {
machineState = ST_R;
}
}
And don’t forget to actually bind the interrupt function in setup():
attachInterrupt(digitalPinToInterrupt(BUTTON), ISR_ButtonToggle, FALLING);
Now that’s a simple and pretty useless traffic light (well, I still like that I can switch it to green at will, this really should be the default pedestrian crossing light!).
But it is easy to extend. You want a yellow state? Add a yellow state ST_Y to your state machine (the switch case statement). Or you want it to show yellow before switching from green to red? Add a yellow-before-red state ST_Y_R. Or you really like to let people wait? Add a wait state. Oh, and of course you can call your states whatever you want.
Here is my full implementation of a non-pedestrian red-yellow-green traffic light as it is stated in the German traffic rules:
- From red to green, with wait periods, go through
- Red
- Red+Yellow (yes, red+yellow, yellow meaning “get ready” and red meaning “not too fast, it’s still red, Freundchen!”)
- Green
- From green to red, with wait periods, go through
- Green
- Yellow (yes, only yellow. The meaning is disputed. Reasonable people say it means “Prepare to stop, if you can’t make it in time, it’s okay.” while idiots say it means “STEP ON THE GAS!”)
- Red (This is also sometimes disputed, I won’t go into this.)
#include <Arduino.h>
#define BAUDRATE 9600
#define BUTTON DD2
#define LED_RED DD3
#define LED_YELLOW DD4
#define LED_GREEN DD5
// Transition delays
#define BLINK_WAIT 7
#define DELAY_R_RY 250
#define DELAY_RY_G 250
#define DELAY_G_Y 250
#define DELAY_Y_R 250
#define DELAY_R_G 250
#define DELAY_G_R 250
// States
enum states{ ST_R,
ST_RY,
ST_G,
ST_Y,
WAIT_R_RY,
WAIT_RY_G,
WAIT_G_Y,
WAIT_Y_R,
WAIT_G_R,
WAIT_R_G };
states machineState = ST_R;
bool builtin_led = false;
void setState(bool red, bool yellow, bool green) {
digitalWrite(LED_RED, red ? HIGH : LOW);
digitalWrite(LED_YELLOW, yellow ? HIGH : LOW);
digitalWrite(LED_GREEN, green ? HIGH : LOW);
}
void setRed() { setState(true, false, false); }
void setRedYellow() { setState(true, true, false); }
void setYellow() { setState(false, true, false); }
void setGreen() { setState(false, false, true); }
void blinkDelay(int delay_ms) {
for(int i=0; i<BLINK_WAIT; i++){
delay(delay_ms);
digitalWrite(LED_BUILTIN, builtin_led);
Serial.write(".");
builtin_led = !builtin_led;
}
Serial.write("\n");
}
void ISR_ButtonToggle() {
Serial.write("BUUUUTTON!\n");
if(machineState == ST_R) {
machineState = WAIT_R_RY;
} else if (machineState == ST_G) {
machineState = WAIT_G_Y;
}
}
void setup() {
pinMode(LED_BUILTIN, OUTPUT);
pinMode(LED_RED, OUTPUT);
pinMode(LED_YELLOW, OUTPUT);
pinMode(LED_GREEN, OUTPUT);
digitalWrite(LED_BUILTIN, LOW);
digitalWrite(LED_RED, LOW);
digitalWrite(LED_YELLOW, LOW);
digitalWrite(LED_GREEN, LOW);
pinMode(BUTTON, INPUT);
attachInterrupt(digitalPinToInterrupt(BUTTON), ISR_ButtonToggle, FALLING);
Serial.begin(BAUDRATE);
Serial.write("Ready.\n");
}
void loop() {
switch(machineState) {
case ST_R:
// Serial.write("State: Red");
setRed();
break;
case ST_G:
// Serial.write("State: Green");
setGreen();
break;
case WAIT_R_RY:
Serial.write("Wait: Red->RedYellow\n");
blinkDelay(DELAY_R_RY);
machineState = ST_RY;
break;
case ST_RY:
setRedYellow();
machineState = WAIT_RY_G;
break;
case WAIT_RY_G:
Serial.write("Wait: RedYellow->Green\n");
blinkDelay(DELAY_RY_G);
machineState = ST_G;
break;
case WAIT_G_Y:
Serial.write("Wait: Green->Yellow\n");
blinkDelay(DELAY_G_Y);
machineState = ST_Y;
break;
case ST_Y:
setYellow();
machineState = WAIT_Y_R;
break;
case WAIT_Y_R:
Serial.write("Wait: Yellow->Red\n");
blinkDelay(DELAY_Y_R);
machineState = ST_R;
break;
case WAIT_R_G:
Serial.write("Wait: Red->Green\n");
blinkDelay(DELAY_R_G);
machineState = ST_G;
break;
case WAIT_G_R:
Serial.write("Wait: Green->Red");
blinkDelay(DELAY_G_R);
machineState = ST_R;
break;
default:
Serial.write("Woops...\n");
machineState = ST_R;
}
}
Is this the best way to write this? Hell, no! Is it even a good tutorial? You tell me. I think it’s fairly simple and shows some evenly common concepts:
- Using IO pins
- Wiring LEDs without burning them or the output pin
- Wiring a button*
- Using #define statements to make code more readable and lives more easy
- Using some abstraction (setState(), setRed(), etc.)
- Using a state machine as an extensible concept
- Using an interrupt (Why use an interrupt? Because it interrupts your code exactly when the button is pressed. It makes your little circuit very responsive and – at least in this example – it is even easier to write.)
*) You may encounter a problem that goes by the name “bouncing” (or in the beautiful German language “prellen”). That’s when your button press toggles multiple interrupts in a row. Try putting a capacitor in parallel with the resistor, or as a bad software hack, add a 5 ms delay (delay(5);) to the end of your interrupt function.
Anyways: My girl loves it. Ha! You thought she’d be a boy because it was a traffic light. Shame on you!